TypeScript SDK
Developer Tools for Meeting Automation
The TypeScript SDK is the officially supported package that empowers developers to integrate with the Meeting BaaS API - the universal interface for automating meetings across Google Meet, Zoom, and Microsoft Teams.
- Complete type safety with comprehensive TypeScript definitions
- Automatic updates synced with our OpenAPI specification
- Simplified access to all meeting automation capabilities
- Cross-platform consistency for all supported meeting providers
Key Features
- BaaS API Client: Strongly typed functions for interacting with the Meeting BaaS API
- Bot Management: Create, join, and manage meeting bots across platforms
- Calendar Integration: Connect calendars and schedule meeting recordings
- Complete API Coverage: Access to all Meeting BaaS API endpoints
- TypeScript Support: Full TypeScript definitions for all APIs
- MPC Tool Integration: Pre-generated MPC tools for AI systems
- CLI Interface: Command-line tools for common operations
- Automatic Tool Generation: MPC tools automatically generated for all SDK methods
Installation
Install the Meeting BaaS SDK using your preferred package manager:
Use npm to install the SDK package:
npm install @meeting-baas/sdk
Quick Start
Create a new instance of the BaaS client with your API key:
This TypeScript example shows basic client initialization and bot deployment:
// SDK Example: Join a meeting with a bot import { BaasClient } from '@meeting-baas/sdk'; // Initialize client const client = new BaasClient({ apiKey: 'your-api-key', baseUrl: 'https://api.meetingbaas.com' }); // Join a meeting with a bot async function joinMeeting() { try { const botId = await client.join({ joinRequest: { meetingUrl: 'https://meet.google.com/abc-def-ghi', botName: 'Meeting Assistant', reserved: true } }); console.log('Bot created:', botId); } catch (error) { console.error('Failed to join:', error); } }
Calendar Integration
The SDK provides full calendar integration capabilities:
This example demonstrates how to interact with calendars using the API:
import json from datetime import datetime, timedelta import requests # Your API key api_key = "your-meetingbaas-api-key" # API endpoints base_url = "https://api.meetingbaas.com" calendars_url = f"{base_url}/calendars" # Headers for authentication headers = {"Content-Type": "application/json", "x-spoke-api-key": api_key} # 1. List connected calendar providers def list_calendar_providers(): response = requests.get(f"{calendars_url}/providers", headers=headers) return response.json() # 2. Schedule bots for all team meetings in the next week def schedule_bots_for_team_meetings(): # Calculate date range (next 7 days) today = datetime.now().isoformat() next_week = (datetime.now() + timedelta(days=7)).isoformat() # Query parameters to find team meetings params = {"start_time": today, "end_time": next_week, "query": "Team Meeting"} # Get matching events events_response = requests.get( f"{calendars_url}/events", headers=headers, params=params ) if events_response.status_code != 200: return f"Error fetching events: {events_response.text}" events = events_response.json().get("events", []) scheduled_bots = [] # Schedule a bot for each meeting for event in events: bot_data = { "meeting_url": event.get("meeting_url"), "scheduled_time": event.get("start_time"), "bot_name": "Team Meeting Recorder", "speech_to_text": "Gladia", "entry_message": "I'll be recording this team meeting.", } bot_response = requests.post(f"{base_url}/bots", headers=headers, json=bot_data) if bot_response.status_code == 200: bot = bot_response.json() scheduled_bots.append( { "event_id": event.get("id"), "event_title": event.get("title"), "bot_id": bot.get("bot_id"), } ) return {"total_events": len(events), "scheduled_bots": scheduled_bots} # Run the example if __name__ == "__main__": providers = list_calendar_providers() print(f"Connected calendar providers: {providers}") result = schedule_bots_for_team_meetings() print(json.dumps(result, indent=2))
MPC Tools Integration
The SDK includes pre-generated MPC (Model Context Protocol) tools that can be easily integrated with any MPC server implementation:
The following JSON configuration shows how to configure MPC tools for Meeting BaaS:
{ "mcpServers": { "MeetingBaasDocs": { "url": "https://mcp.meetingbaas.com/sse", "env": { "BAAS_URL": "https://api.meetingbaas.com", "REDIS_URL": "rediss://default:AZXXXXXXXX32.upstash.io:6379" } } } }
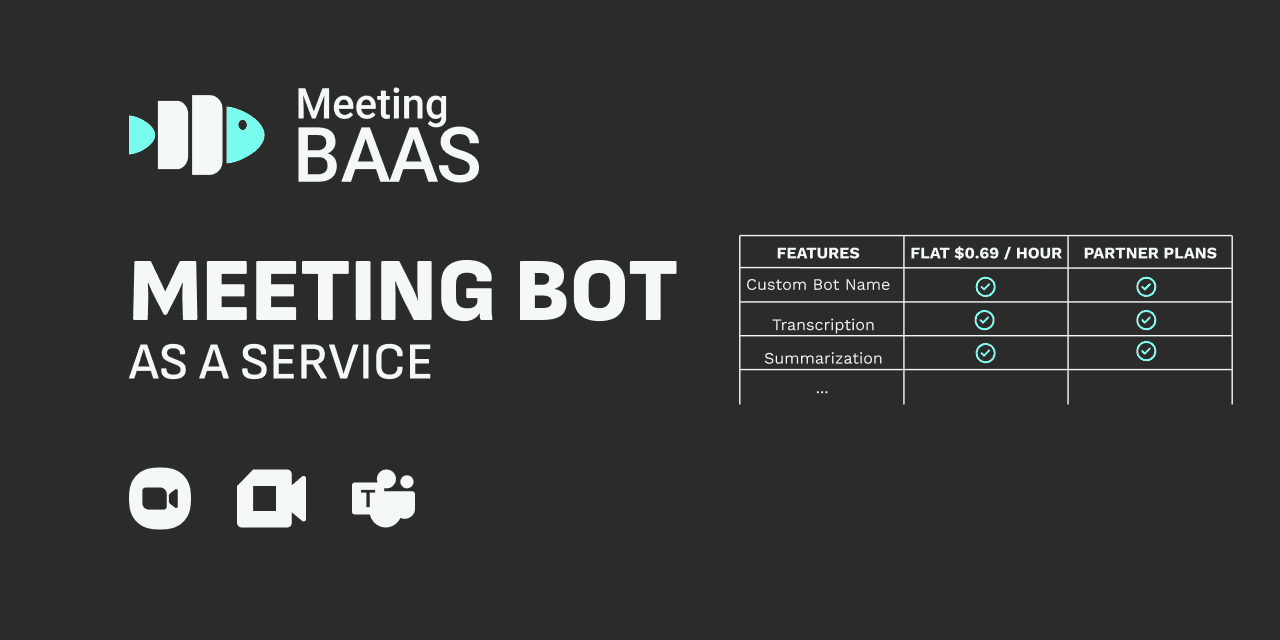
Resources
Open-Source
Transcript SeekerTranscript Seeker: a complete interface in ReactSpeaking Bots: AI-powered meeting participantsMCP Tools - Model Context ProtocolMeeting MCP ServerMCP on VercelMCP Documentation ServerNode server for Notion summariesSimple Transcript player in ReactMeeting summarization using Make.comInterface for the Gladia transcription APIInterface for the AssemblyAI transcription API
APIs
Product
PlansFeature ListMeeting Transcription APIAI Meeting Assistant APIAPI to Record and Transcribe Video MeetingsMeeting bots with transcriptionMeeting bot API for Google MeetAlternative Recording API for Google MeetAlternatives to Recall AIRecall AI vs Meeting BaaSMeeting bot API for ZoomMeeting bot API for Microsoft Teams