Calendars API
Synchronize Calendars and Automate Meeting Recordings
The Calendars API allows you to automatically sync calendars from Outlook and Google Workspace to deploy bots to scheduled meetings.
This makes it easy to:
- Automate recording and participation in meetings without manual intervention
- Connect to both Google Workspace and Microsoft Outlook calendars
- Receive real-time updates when calendar events change
- Apply business logic to determine which meetings to record
The API follows a simple pattern allowing you to connect calendar providers, list and manage events, and automatically schedule recording bots when meetings occur.
Key Features
- Multi-Calendar Support: Connect to both Google Workspace and Microsoft Outlook calendars
- OAuth Authentication: Secure integration with calendar providers using OAuth
- Real-Time Webhooks: Receive immediate notifications when calendar events change
- Selective Recording: Implement your own business rules to decide which meetings to record
- Bot Configuration: Customize recording settings for each meeting
- Recurring Meeting Support: Handle recurring meeting series efficiently
Implementation Process
- Authentication Setup: Connect calendars using OAuth credentials
- Calendar Selection: Choose which calendars to sync
- Event Management: List, filter, and process calendar events
- Recording Configuration: Schedule bots for specific meetings
- Webhook Processing: Receive and handle real-time calendar updates
- Maintenance: Handle credential refreshing and error recovery
API Usage
The Calendars API is part of the Meeting BaaS platform and provides endpoints for:
- Creating and managing calendar integrations
- Listing and filtering calendar events
- Scheduling recording bots for specific meetings
- Managing webhooks for real-time updates
The API is fully documented and supports standard REST operations with JSON payloads. Authentication is performed using your Meeting BaaS API key along with OAuth tokens for calendar provider access.
API Usage Example
Below is an example of how to use the Calendars API to schedule bots for team meetings:
This code demonstrates how to interact with the Calendars API to retrieve events and schedule recording bots.
import { createBaasClient } from "@meeting-baas/sdk";
const client = createBaasClient({
api_key: "your-api-key",
timeout: 30000 // optional, default: 30000
});
const { success, data, error } = await client.createCalendar({
oauth_client_id: "your-oauth-client-id",
oauth_client_secret: "your-oauth-client-secret",
oauth_refresh_token: "your-oauth-refresh-token",
platform: "Google",
raw_calendar_id: "optional-calendar-id"
});
if (success) {
console.log("Calendar created:", data.calendar.name);
console.log("Calendar UUID:", data.calendar.uuid);
} else {
console.error("Error creating calendar:", error);
}
curl -X POST "https://api.meetingbaas.com/calendars/" \
-H "x-meeting-baas-api-key: <token>" \
-H "Content-Type: application/json" \
-d '{
"oauth_client_id": "string",
"oauth_client_secret": "string",
"oauth_refresh_token": "string",
"platform": "Google",
"raw_calendar_id": "string"
}'
List Calendar Events
Here's how to retrieve events from a connected calendar:
import { createBaasClient } from "@meeting-baas/sdk";
const client = createBaasClient({
api_key: "your-api-key"
});
// List events from a specific calendar
const { success, data, error } = await client.listCalendarEvents({
calendar_uuid: "your-calendar-uuid",
start_time: "2024-01-01T00:00:00Z",
end_time: "2024-01-31T23:59:59Z"
});
if (success) {
console.log("Events found:", data.events.length);
data.events.forEach(event => {
console.log(`- ${event.summary} (${event.start_time})`);
});
} else {
console.error("Error listing events:", error);
}
Schedule Recording for Events
Automatically schedule bots for upcoming meetings:
import { createBaasClient } from "@meeting-baas/sdk";
const client = createBaasClient({
api_key: "your-api-key"
});
// Schedule recording for a specific calendar event
const { success, data, error } = await client.scheduleRecording({
calendar_uuid: "your-calendar-uuid",
event_id: "event-id-from-calendar",
bot_config: {
bot_name: "Meeting Recorder",
recording_mode: "speaker_view",
speech_to_text: {
provider: "Default"
}
}
});
if (success) {
console.log("Recording scheduled:", data.scheduled_recording.id);
} else {
console.error("Error scheduling recording:", error);
}
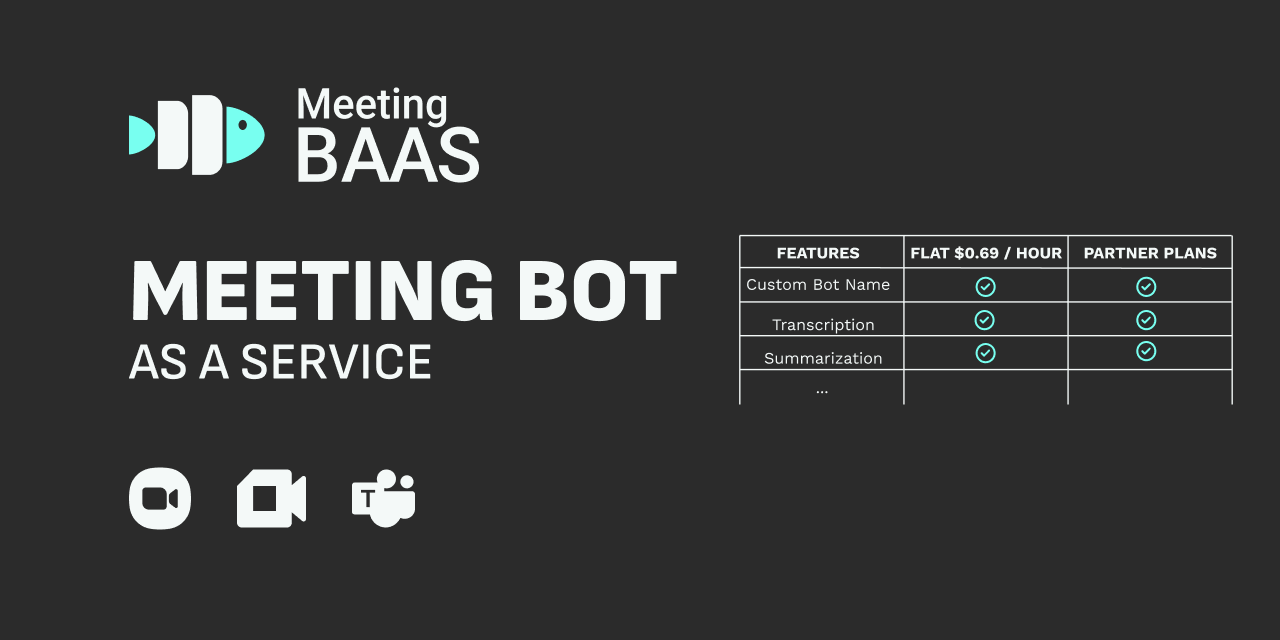
Get Started
Ready to automate your meeting recordings with calendar integration? Check out our comprehensive documentation: